Create a Log Exceptions in MVC
To Log Exceptions in a .NET Application, you should follow the below steps in details:
Create a static class Named LogMiddleware
Create Static Method Named LogError with parameters ( Exception ex , string MethodName )
Invoke this method at any location within your application .
Code:
public static void LogError(Exception ex, string MethodName)
{
string logPath = ConfigurationManager.AppSettings["LogPath"];
string filePath = logPath + DateTime.Now.ToString("yyyy-MM-dd") + ".txt";
string ErrorlineNo, Errormsg, extype, exurl, ErrorLocation;
var line = Environment.NewLine + Environment.NewLine;
ErrorlineNo = ex.StackTrace.Substring(ex.StackTrace.Length - 7, 7);
Errormsg = ex.GetType().Name.ToString();
extype = ex.GetType().ToString();
exurl = HttpContext.Current.Request.Url.ToString();
ErrorLocation = ex.Message.ToString();
try
{
logPath = logPath + DateTime.Today.ToString("yyyy-MM-dd") + ".txt"; //Text File Name
if (!File.Exists(logPath))
{
File.Create(logPath).Dispose();
}
using (StreamWriter sw = File.AppendText(logPath))
{
string error = "Log Written Date:" + " " + DateTime.Now.ToString() + line +
"Error Line No :" + " " + ErrorlineNo + line +
"Error Message:" + " " + Errormsg + line +
"Exception Type:" + " " + extype + line +
"Error Location :" + " " + ErrorLocation + line +
"Error Page Url:" + " " + exurl + line +
"StackTrace:" + " " + ex.StackTrace;
sw.WriteLine("-----------Exception Details on " + " " + DateTime.Now.ToString() + "-----------------");
sw.WriteLine("-------------------------------------------------------------------------------------");
sw.WriteLine(line);
sw.WriteLine(error);
sw.WriteLine(line);
sw.WriteLine("MethodName :" + MethodName);
sw.WriteLine("--------------------------------*End*------------------------------------------");
sw.WriteLine(line);
sw.Flush();
sw.Close();
}
}
catch (Exception e)
{
e.ToString();
}
}
LogPath: Directory on the machine where exception files are deposited.
Result:
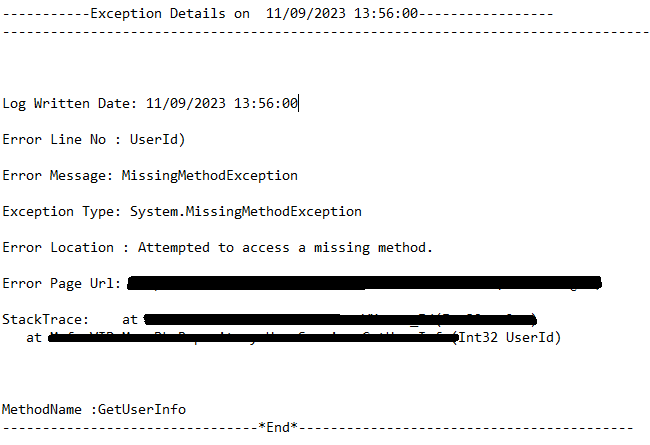